To use cookies in Node Express JS, we will have to import cookie-parser middleware. For installation of cookie-parser, execute the following command on your terminal.
# npm install --save cookie-parser
How to set cookies in Express JS?
To start using cookies, we will import
//imports express module
let express = require('express');
//initializes express app
let app = express();
//initialize cookieparser
let cookieParser = require('cookie-parser');
//sets template engine
app.set('view engine', 'pug');
//sets directory name
app.set('views','./views');
//use cookie parser
app.use(cookieParser());
//use middleware to serve static files
app.use('/static', express.static('public'));
app.get('/', function(request, response){
//the following line sets cookies on client side
response.cookie('username','LearnCyber').send('Username: LearnCyber');
});
//listens to server at port 3000
app.listen(3000);
Look at the above code, we have imported cookie-parser and initialized it with
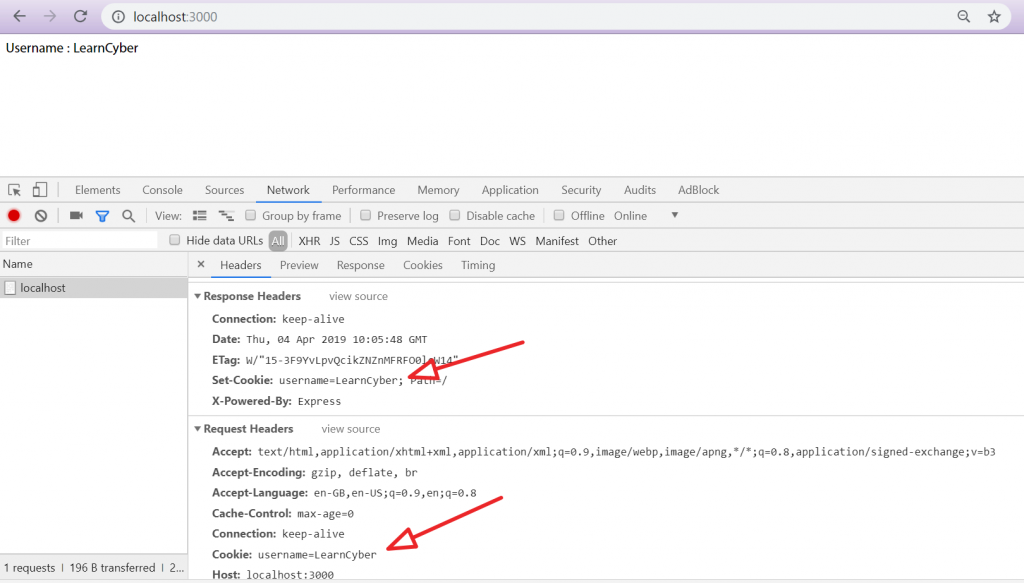
How to set cookies with expiry headers to speed up your website?
Search Engine Optimization (SEO) is considered to be most important part of any commercial web application or blog. Most of the search engines also consider speed as important factor to rank a website. To speed up the website loading and enhance user friendliness, we will add expiry time of cookies. There are two ways to set expiry time for cookies. One is to use absolute time and other is to use relative time.
We can define
//imports express module
let express = require('express');
//initializes express app
let app = express();
//initialize cookieparser
let cookieParser = require('cookie-parser');
//sets template engine
app.set('view engine', 'pug');
//sets directory name
app.set('views','./views');
//use cookie parser
app.use(cookieParser());
//use middleware to serve static files
app.use('/static', express.static('public'));
app.get('/', function(request, response){
//sets cookie with relative expiry time
response.cookie(username1, 'Learn', {maxAge: 480000});
//sets cookie with absolute expiry time
response.cookie(username2, 'Cyber', {expire: 480000 + Date.now()});
});
//listens to server at port 3000
app.listen(3000);
How to delete cookies in Express JS?
We can also delete cookies to avoid tracking and information about customization by using clearCookie(‘name’) function as shown below.
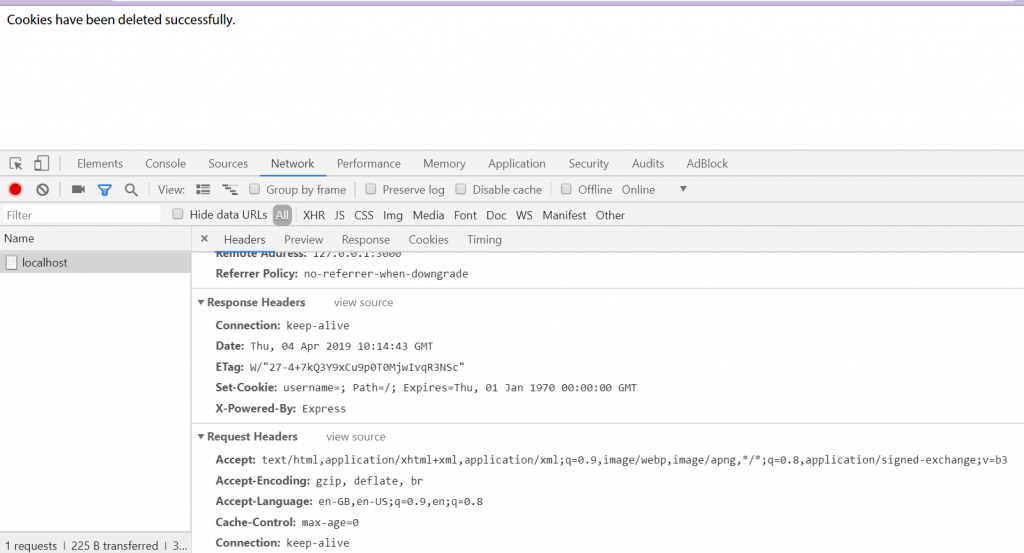