Table of Contents
Factory Design Pattern:
A Factory Design Pattern or Factory Method Pattern says that it only defines an interface or abstract class that creates an object. Subclasses are therefore responsible for creating the class case.
Factory Design Method is also known as Virtual Constructor.
Advantages of Factory Design Pattern:
- The factory design method allows sub-classes to choose the object type that they create.
- This promotes loose connection by eliminating the need for binding specific application classes to the code. It means that the code only communicates with the resulting interface or abstract class, so it operates with all classes that enforce or extend it, abstract class.
Usage of Factory Design pattern:
- If a class doesn’t understand what subclasses are needed to create.
- If a class needs to define the objects to be generated in its subclasses.
- If the parent classes choose to create objects in their subclasses.
Deployment:
We will create a plan that extends the Plan abstract class and concrete classes. The next move is to identify a GetPlanFactory factory class.
GetPlanFactory will be used to acquire a Project object in the GenerateBill package. It will transmit data to the GetPalnFactory to get the kind of object it needs. (DOMESTERS / COMMIRCIALPLAN / INSTITUTIONALPLEN).
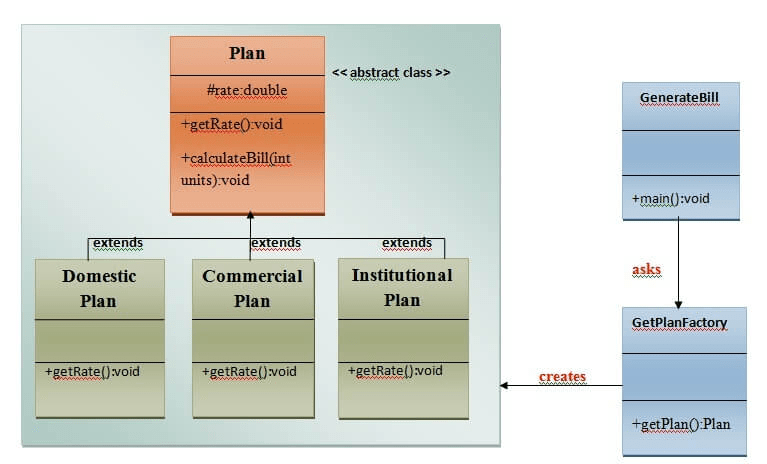
Electricity Bill Calculate: A Global Factory Method Sample:
Phase-1: Construct an explicit class plan.
import java.io.*.;
abstract class Plan {
Protected double rate;
abstract void getRate();
public void calculateBill(int units){
System.out.println(units*rate);
}
}//end of Plan class.
Phase-2: Creating concrete classes to cover the abstract class of Plan.
class DomesticPlan extends Plan{
//@override
public void getRate(){
rate=3.50;
}
}//end of DomesticPlan class.
class CommercialPlan extends Plan{
//@override
public void getRate(){
rate=7.50;
}
/end of CommercialPlan class.
class InstitutionalPlan extends Plan{
//@override
public void getRate(){
rate=5.50;
}
/end of InstitutionalPlan class.
Phase-3: Build a GetPlanFactory to create artefacts of specific classes based on data.
class GetPlanFactory{
//use getPlan method to get object of type Plan
public Plan getPlan(String planType){
if(planType == null){
return null;
}
if(planType.equalsIgnoreCase("DOMESTICPLAN")) {
return new DomesticPlan();
}
else if(planType.equalsIgnoreCase("COMMERCIALPLAN")){
return new CommercialPlan();
}
else if(planType.equalsIgnoreCase("INSTITUTIONALPLAN")) {
return new InstitutionalPlan();
}
return null;
}
}//end of GetPlanFactory class.
Phase-4: Generate the Bill by use of the GetPlanFactory to transmit data such as the DOMESTICPLAN, COMMERCIALPLAN or INSTITUTIONALPLAN plan form to the target in specific classes.
import java.io.*;
class GenerateBill{
public static void main(String args[])throws IOException{
GetPlanFactory planFactory = new GetPlanFactory();
System.out.print("Enter the name of plan for which the bill will be generated: ");
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String planName=br.readLine();
System.out.print("Enter the number of units for bill will be calculated: ");
int units=Integer.parseInt(br.readLine());
Plan p = planFactory.getPlan(planName);
//call getRate() method and calculateBill()method of DomesticPaln.
System.out.print("Bill amount for "+planName+" of "+units+" units is: ");
p.getRate();
p.calculateBill(units);
}
}//end of GenerateBill class.
Output:
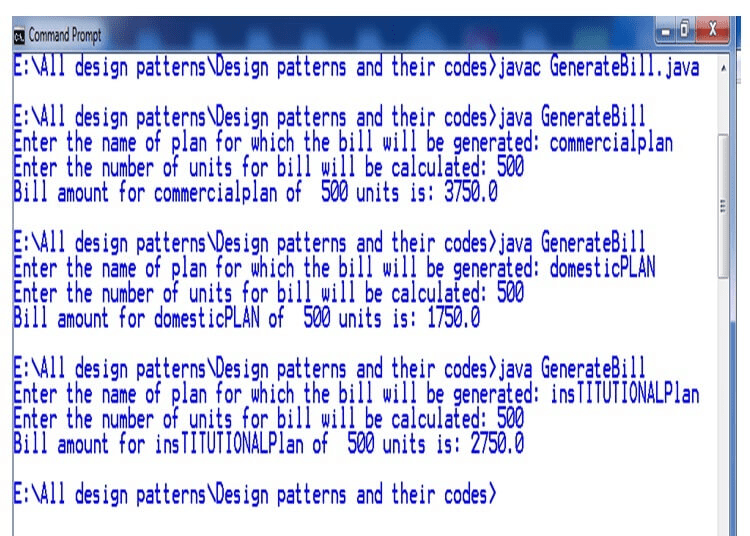
You may be interested in reading about Machine Learning.