Express Middleware comes between request and response. When a request is made, it is received by the server where middleware performs its tasks on the received request and then route handler sends the response which again passes through middleware functions and in the end reaches to the client.
These functions have access to requests, responses and next middleware function in
For example, if you want to get a date at each request made to the server, you will need generic middleware rather than route specific. Example code is given below.
//imports express module
let express = require('express');
//initializes express app
let app = express();
//request response cycle
app.use(function(req, res, next){
console.log("A request has been received at " + Date.now());
//this function tells us that more processing is needed for current
//request and next middleware.
next();
});
//listens to server at port 3000
app.listen(3000);
Whenever a request is made to any route, it will give the date and time for the request made.
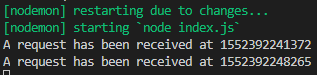
To make it route specific, we will add
//imports express module
let express = require('express');
//initializes express app
let app = express();
//request response cycle
app.use('/', function(req, res, next){
console.log("A request has been received at " + Date.now());
//this function tells us that more processing is needed for current request and next middleware.
next();
});
//request handler for sending response
app.get('/', function(req, res){
res.send('Success!');
});
//listens to server at port 3000
app.listen(3000);
Express Middleware Calls Order in Node Express JS
Middleware functions are executed in
//imports express module
let express = require('express');
//initializes express app
let app = express();
//request response cycle
app.use('/', function(req, res, next){
console.log("A request has been received at " + Date.now());
//this function tells us that more processing is needed for current request and next middleware.
next();
});
//request handler for sending response
app.get('/', function(req, res, next){
res.send('Success!');
next();
});
app.use('/', function(req, res){
console.log('Ended successfully!')
});
//listens to server at port 3000
app.listen(3000);
app.use() will execute, then app.get() will execute and in the end app.use() will execute and print Ended successfully! on console. After accessing http://localhost:3000/, the following output gets printed on console.

Express Middleware developed by 3rd Party
Express JS supports middleware provided by
Some 3rd Party Middleware compatible with Express JS
body-parser | This middleware helps in parsing HTTP request body. |
compression | This middleware helps in compressing HTTP responses. |
cookie-parser | This middleware helps in parsing cookie header to populate request.cookies. |
csurf | This middleware helps in protecting from CSRF exploits. |
multer | It is used when we have to handle multi-part form for uploading files. |
session | This middleware is used in establishing server-based sessions. Mostly it is used in development only because in production we prefer to use cookie based sessions. |
response-time | This records HTTP response time which helps us in improving our application. |
Each of the middleware is installed by executing command.
$ npm install --save <middleware name>

To use any, you will import it in your index.js file and the use app.use() method. For example, cookie-parser can be used as:
//imports express module
let express = require('express');
//initializes express app
let app = express();
let cParser = require('cookie-parser');
app.use(cParser())
//listens to server at port 3000
app.listen(3000);
In this tutorial, you have learnt about Express Middleware. In the next tutorial, you will learn about Frontend Templating in Express JS using Pug Engine.