Express router helps in defining URL for web applications and APIs. It makes easier to create SEO friendly URLs.
What is express router?
When we are dealing with complex applications which needs a big list of routes, it becomes difficult to handle everything in single index.js file. To avoid this, we can use Express.Router and create a separate file named routes.js in the same directory where index.js exists. Now add the following code in your routes.js file. All the express router routes will be added in routes.js file.
var express = require('express');
//initialize router
var router = express.Router();
//get request
router.get('/home', function(request, response){
response.send("Welcome to GET");
});
//post request
router.post('/home', function(request, response){
response.send("Welcome to POST");
});
//it enables to include this file's code in index.js file
module.exports = router;
Update your index.js as below:
//imports express module
let express = require('express');
//initializes express app
let app = express();
//import routes
let routes = require('./routes.js');
app.use('/routes', routes);
//listens to server at port 3000
app.listen(3000);
Now you will not be able to access directly http://localhost:3000/home. To access home, you will need to route through /routes
Look at one thing, you can access home but cannot pass any value to it.
We have only studied static routing in Express JS yet. Now we will move towards learning Dynamic Routing in Express JS which will help us in building URLs as per our requirements.
Dynamic Routing and URL Building in Express JS
Dynamic Routing allows us to pass parameters and values in routes on the basis of which the server processes our request and sends back the response. This is not fixed or static. The value of
For understanding purpose, let’s see Facebook profile link https://fb.com/itsaareez1 where itsaareez1 is profile’s username. Every user has a unique username so, this value will vary according to
//imports express module
let express = require('express');
//initializes express app
let app = express();
//where username is variable starting with colon
app.get('profile/:username', function(request, response){
response.send('Welcome to ' + request.params.username + ' profile.');
});
//listens to server at port 3000
app.listen(3000);
You can see I have accessed http://localhost:3000/profile/itsaareez1 and received response Welcome to itsaareez1 profile.


Now let’s pass BestCyberHacks as value, you will see the result as below.


You can also pass multiple parameters to URL in Express JS. The following code takes username and post id as
//imports express module
let express = require('express');
//initializes express app
let app = express();
//where username is variable starting with colon
app.get('/profile/:username/:post_id', function(request, response){
response.send('Welcome to ' + request.params.username + ' profile. We are fetching post_id'
+ request.params.post_id);
});
//listens to server at port 3000
app.listen(3000);
The output of above code is given below.


In the above examples, we have used request.params.parameter_name to access the given value where parameter_name is replaced with original parameter name.
Pattern Matched Routes in Express JS
Express router also supports pattern matching in its routing to restrict the values passed to URL parameter. For example, if you want to take
//imports express module
let express = require('express');
//initializes express app
let app = express();
//where username is variable starting with colon
app.get('/profile/:username/:post_id([0-9]{2})', function(request, response){
response.send('Welcome to ' + request.params.username + ' profile. We are fetching post_id'
+ request.params.post_id);
});
//listens to server at port 3000
app.listen(3000);
Now I have accessed http://localhost:3000/profile/BestCyberHacks/54, it worked fine as shown below.


But when I have changed


As only numeric value is acceptable, when I used the link http://localhost:3000/profile/BestCyberHacks/a4, it resulted in the following error.


A user can access the URL in many ways. An application has limited number of routes defined but there are unlimited possibilities that a user can enter to access. To avoid the requests for invalid routes, we may use * as route. The code is shown below.
//imports express module
let express = require('express');
//initializes express app
let app = express();
//home route
app.get('/home', function(request, response){
response.send('Good response!');
});
//where username is variable starting with colon
app.get('*', function(request, response){
response.send('Invalid URL');
});
//listens to server at port 3000
app.listen(3000);
Now when you will access
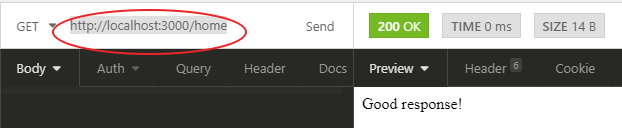
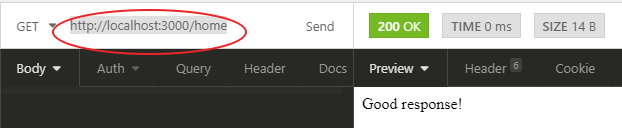
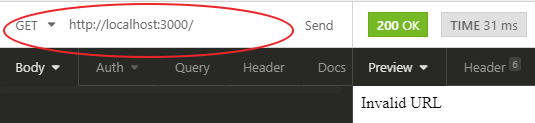
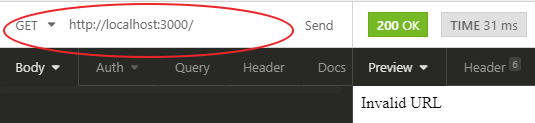
In this tutorial, you have learnt about Express routerIn the next tutorial, you will learn about middleware in Express JS.